Universal Content Picker
The Seismic Content Picker control allows developers to add support for choosing files and folders from Library, Workspace, DocCenter, Recents and Favorites from any web application. The picker handles all communication with the Seismic APIs and returns information about the files selected to the containing application via a JavaScript callback.
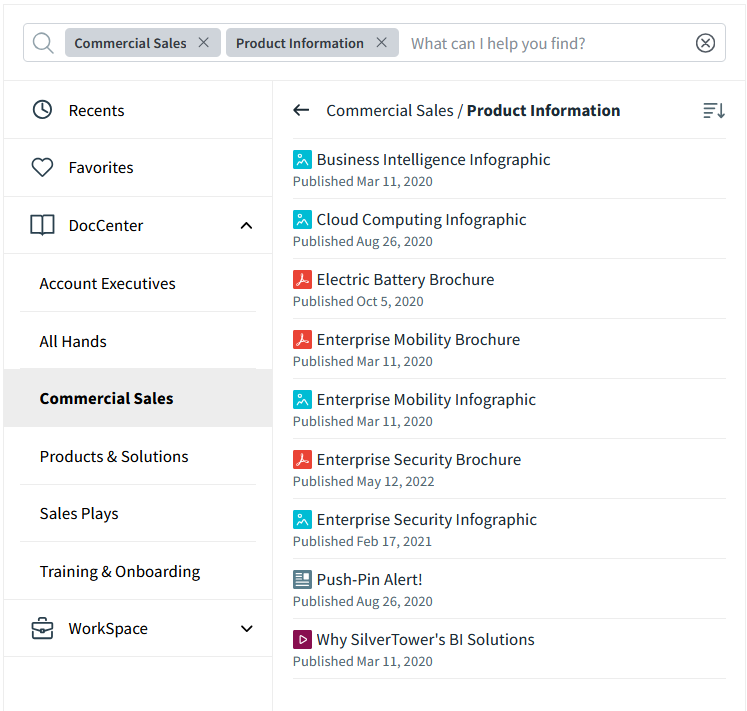
Purpose
Seismic's Content Picker, frequently referred to as the Universal Content Picker, is a component that allows you to see and interact with your seismic content in an efficient and lightweight client that is designed to be embedded in other applications. UCP is the foundation of many existing integrations, both those owned by Seismic and others created by clients and 3rd parties.
Common use cases include:
- Inserting a LiveSend link into a 3rd party platform, such as Outlook, Gmail, Salesloft, or Outreach
- Surfacing Seismic content search into your intranet or other highly used platforms
- Workspace and Contextual Workspace folders for easy content access in your CRMs or Marketing Automation tools
Browser Support
Full Support:
- Chrome
- Chrome mobile
- Edge
- Firefox
- Safari
- Safari mobile
- Mobile Chrome and Safari
Limited support
- Internet Explorer 11 (requires ES2015/Intl polyfill)
Security & Authentication
The Content Picker needs permissions to access your Seismic content which is achieved by requesting an authentication token through your Seismic Hosted application. If you are unfamiliar with this concept, you can read more about it here: Authentication - high level & demo
Which scopes should I use in my token?
When requesting a token, you will need to request access the following scopes, and your app will also need to be configured to allow these specific scopes. You can read more about Scopes here: Scopes
- seismic.self.view
- seismic.search
Getting started - Developer details
To quickly load a Seismic Platform component, you first must include the Seismic Bootstrapper script in the DOM and create an empty container element for the component to live in. The bootstrapper will check the containing application for dependencies already loaded, like react and only load what isn't already available.
Updated seismic-bootstrapper to version 2.0.0
Please refer to the updated HTML script below
<script crossorigin src="https://cdn-prod.seismic.com/static/public/seismic-bootstrapper/2.0.0/SeismicBootstrapper.js"></script>
<div id="contentPickerContainer"></div>
After the script is loaded and the container element in place, you must initialize the Bootstrapper and then attach the component to the element.
Updated content picker to version 6
Please refer to the updated Javascript below
const options = {
manifestPath: "https://cdn-prod.seismic.com/static/public/",
token: ''
};
const containerEl = document.getElementById('contentPickerContainer');
window.SeismicPlatform.SeismicBootstrapper.init(options);
function tokenResolver() {
return options.token
};
function apiDomainResolver() {
return "api.seismic.com"
};
function headerResolver() {
return {
'Authorization': 'Bearer ' + tokenResolver(),
'Content-Type': 'application/json',
}
};
const apiOptions = {
apiDomainResolver: apiDomainResolver,
authTokenResolver: tokenResolver,
globalErrorHandlers: {},
customHeadersResolver: headerResolver,
};
window.SeismicPlatform.SeismicBootstrapper.attach("universal-content-picker@6", {
settings: {
"language": "en-US",
"selectionMode": "single",
"allowFolderSelection": false,
"repositories": ["recents", "favorites", "doccenter", "workspace", "library"],
"initialFolderId": {},
"viewMode": "narrow",
"contentTypeFilter": [],
"hideContentTypeFilteredItems": true,
"deliveryOptionsFilter": [],
"hideDeliveryOptionsFilteredItems": true
},
apiOptions: apiOptions,
callbacks: {
onSelectionChanged: function (items) {
console.log(items);
},
onPreviewClick: function (item) {
console.log(items);
}
}
}, containerEl);
Options
In addition to the options listed above, the following options can be used to customize the Seismic Content Picker for your use case.
Name | IsRequired | Value Range |
---|---|---|
language | N (default to en-US) | de-DE, en-GB, en-US, es-ES, fr-CA, fr-FR, it-IT, ja-JP, ko-KR, pt-BR, zh-CN, zh-TW, el-CY, tr-CY |
selectionMode | Y | multi: Multiple contents can be selected single: Only single document can be selected drag: Contents can be dragged outside of UCP and selected by dropping on drag target |
allowFolderSelection | N (default to true) | true: Folders can be selected false: Folder can’t be selected |
contentTypeFilter | N | "PPTX","PPT", "Email Template", etc... |
hideContentTypeFilteredItems | N | true: Hide items not matching with the filter false: Gray out items not matching with the filter |
deliveryOptionsFilter | N | "Livesend","Generate Livesend Link" |
hideDeliveryOptionsFilteredItems | N | true: Hide items not matching with the filter false: Gray out items not matching with the filter |
viewMode | Y | narrow: Load in narrow view design. wide: Loads in wide view |
repositories | N | "recents", "favorites", "doccenter", "workspace", "library" |
initialFolderId | N | Content Manager { "repository": "library", "folderId": "", "teamsiteId": ""} NOTE: Enter "folderId": "root" to show all contents in the given teamsite. Workspace {"repository": "workspace", "folderId": ""} DocCenter {"repository": "doccenter", "folderId": "", "contentProfileId": ""} |
assetTypeFilter | N | { "filterType": "blacklist", "values": ["LiveDoc", "LiveDoc Component", "Email Template"] } {"filterType": "whitelist", "values": ["File"] } |
formatFilter | N | { "filterType": "blacklist", "values": ["PPTX"] } {"filterType": "whitelist", "values": ["PNG"] } |
profileFilter | N | profileFilter: { "filterType": "blacklist", "values": ["1fd349ba-78da-4f62-8160-36c0c7c14fa1"] } profileFilter: { "filterType": "whitelist", "values": ["1fd349ba-78da-4f62-8160-36c0c7c14fa1"] } |
showPreview | N (default to false) | true - Adds a preview button items, which on click triggers onPreviewClick callback. |
application | N | The name of your application for reporting purposes |
tintColor | N | # ecf0f1AA, #10c0392b, rgb(170,34,51) |
Example return values
Whenever an item is selected, the picker will execute the function provided to the callbacks.onSelectionChanged
option during initialization, and pass the following JSON to the first parameter.
[
{
"contentTypeNumber": "3",
"selectedFrom": "doccenter",
"repository": "doccenter",
"id": "L2RkMDI5YjIwODctM2VkNC00MDI3LTgzOTAtOThjNmQzYTU5NGIzL2xmYjFkYzBjOGQtN2E3NC00YzE1LTliNTEtMzc2Y2UxYjYyNmRh",
"profileVersionId": "a9642281-04bd-4a37-8bf8-9c70a92be8b1",
"name": "Electric Battery Brochure",
"type": "File",
"format": "PDF",
"description": null,
"parentFolderId": "dd029b2087-3ed4-4027-8390-98c6d3a594b3",
"contentProfileId": "c1309b23-3161-48db-a4b3-42d960dc7902",
"sourceBlobId": "08fec810-8508-4096-b937-3926b980d28f",
"sourceContainerName": "stsenterprise",
"contentProfileName": "Commercial Sales",
"path": [
{
"id": "dd029b2087-3ed4-4027-8390-98c6d3a594b3",
"name": "Product Information"
},
{
"id": "lfb1dc0c8d-7a74-4c15-9b51-376ce1b626da",
"name": "Electric Battery Brochure"
}
],
"thumbnailId": "t0aefa5ec-18df-4de4-a4b7-6544dd0c7571",
"thumbnailUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=ste&c=stsenterprise&id=t0aefa5ec-18df-4de4-a4b7-6544dd0c7571&et=20240731044947&sig=demo%3D",
"sourceBlobDownloadUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=ste&c=stsenterprise&id=08fec810-8508-4096-b937-3926b980d28f&et=20240731044947&fn=08fec810-8508-4096-b937-3926b980d28f.PDF&sig=demo%3D",
"applicationUrls": [
{
"name": "DocCenter Universal Link",
"url": "https://ste.seismic.com/Link/Content/DC6cPb9j8cgqd8FCfmb8gdVmMD9j"
}
],
"libraryContent": {
"id": "f26ad195-d797-41f1-873d-b5358674e2af",
"versionId": "b1dc0c8d-7a74-4c15-9b51-376ce1b626da",
"teamsiteId": "27ec7539-9f13-416f-8262-544309e3df26"
},
"version": "2.0",
"expiresAt": null,
"properties": [
{
"name": "Classification",
"id": "6d42acc4-aacf-0b6e-2117-06a3d03a8841",
"values": [
"External"
]
},
{
"name": "Asset Type",
"id": "d2cbf1fd-bb05-e76e-c417-00ea7dcde25f",
"values": [
"Brochures"
]
},
{
"name": "Industry",
"id": "fed5fcff-51e0-e76c-5529-ca0c3fcba3b6",
"values": [
"CPG",
"Energy",
"Hospitality",
"Manufacturing",
"Technology",
"Telecommunications",
"Retail",
"Entertainment",
"Government",
"Transportation",
"Food & Beverage",
"Education",
"Insurance"
]
},
{
"name": "Products",
"id": "88fab8c7-9b30-e533-41d4-0951c74a0573",
"values": [
"Business Intelligence"
]
},
{
"name": "Sales Stage",
"id": "e6b8a749-a13f-66a3-7dfa-0cdf962191df",
"values": [
"Qualification",
"Presentation & Demo",
"Closed Won",
"Closed Lost"
]
},
{
"name": "Business Outcomes",
"id": "df838a85-7c30-e238-b947-350725fed564",
"values": [
"Reduce Cost",
"Increase Speed to Market"
]
},
{
"name": "Language",
"id": "b11bf6fa-ed86-038f-4bc9-1b023f0c5ade",
"values": [
"French"
]
},
{
"name": "Persona",
"id": "95e73cc6-cb58-8f81-fd33-f04aab732fb6",
"values": [
"IT",
"Marketing",
"Sales",
"C-Level"
]
},
{
"name": "Competitor",
"id": "febb84f0-5484-b875-e3bb-1833d7997931",
"values": [
"Scrotch BI Solutions"
]
},
{
"name": "Sales Tool",
"id": "d429ea60-75c7-a5e2-5d8e-24181dbb4833",
"values": [
"No"
]
},
{
"name": "Top Performer",
"id": "0ac9fb0e-4f45-faf2-dcf8-54403a626f22",
"values": [
"Yes"
]
},
{
"name": "Internal Predicitve",
"id": "f5f20370-6e39-cc21-9cb3-efcd2384959a",
"values": [
"Guided Selling"
]
}
],
"deliveryOptions": [
{
"id": "1756e8d5-dd7c-4921-9d3e-e8d57f402183"
},
{
"id": "4e4d876c-211f-47ec-8f20-687e2ea58dc9"
},
{
"id": "4f289f89-1261-4517-89b2-afa3140eeedd"
},
{
"id": "6656e869-9660-4725-b872-54ceef21bdfa"
},
{
"id": "6686e276-efbd-4393-8c6b-13ddd5b4ee4a"
},
{
"id": "6bf712d9-5e45-4beb-b913-0749372bb757"
},
{
"id": "86dd560e-6cfc-47f0-b571-5377d72d1eb4"
},
{
"id": "d8ddfdaa-5a07-455e-b797-bb12007568e6"
},
{
"id": "da383728-04f1-4eee-a0e1-882be4531df0"
},
{
"id": "ec330dee-13c1-4dbd-8f4a-0777f4bfed16"
}
],
"iconUrl": "https://api.seismic.com/icon-host/v1/content-icons-v2/icon?format=pdf",
"modifiedAt": "2020-10-05T14:10:41.475Z",
"size": 458135,
"urlObjectUrl": null,
"workSpaceVersionType": null,
"majorVersion": 2,
"minorVersion": 0,
"originUrl": null,
"deliveryOptionsDenyList": []
}
]
[
{
"contentTypeNumber": "3",
"selectedFrom": "workspace",
"repository": "workspace",
"id": "9491bc8f-3211-4ddb-9360-0afd34e6c75d",
"userId": "3f8e6093-9dcf-e2b7-bb17-02f8377c6be2",
"name": "Brochure - SaaS Computing",
"type": "File",
"format": "PPTX",
"description": null,
"parentFolderId": "dff7bfff-4bd2-4088-903f-be78662608da",
"sourceBlobId": "40103328-9d5c-46b0-ba04-32e81f134513",
"sourceContainerName": "stsenterprise-collaboration",
"path": [
{
"id": "dff7bfff-4bd2-4088-903f-be78662608da",
"name": "My Files"
},
{
"id": "9491bc8f-3211-4ddb-9360-0afd34e6c75d",
"name": "Brochure - SaaS Computing"
}
],
"thumbnailId": "52443a11-1378-4578-91d3-6a55456dc3e1-C",
"thumbnailUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=ste&c=stsenterprise-collaboration&id=52443a11-1378-4578-91d3-6a55456dc3e1&et=20240731051020&sig=demo%3D",
"sourceBlobDownloadUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=ste&c=stsenterprise-collaboration&id=40103328-9d5c-46b0-ba04-32e81f134513&et=20240731051020&fn=40103328-9d5c-46b0-ba04-32e81f134513.PPTX&sig=demo%3D",
"applicationUrls": [
{
"name": "Default",
"url": "https://ste.seismic.com/share/4ppQFHDEMO"
}
],
"libraryContent": {
"id": "9491bc8f-3211-4ddb-9360-0afd34e6c75d",
"versionId": "4b8b0d77-500b-49e4-912e-91dd6c985ad4",
"teamsiteId": null
},
"versionId": "4b8b0d77-500b-49e4-912e-91dd6c985ad4",
"deliveryOptions": [
{
"id": "4f289f89-1261-4517-89b2-afa3140eeedd"
},
{
"id": "d8ddfdaa-5a07-455e-b797-bb12007568e6"
},
{
"id": "ec330dee-13c1-4dbd-8f4a-0777f4bfed16"
},
{
"id": "403f0842-3628-4524-bd0c-3bddac2d12cc"
},
{
"id": "579f59f3-99c5-46c3-a805-2391ca26b882"
},
{
"id": "c3991a94-5dc2-4488-adc4-46717669aa9c"
},
{
"id": "28d39fce-4a92-4267-a128-a355b1f95c2f"
},
{
"id": "87ff95bc-4bfc-4d08-9c0f-a594acdb6530"
},
{
"id": "6bf712d9-5e45-4beb-b913-0749372bb757"
},
{
"id": "cd448095-c4f9-4a25-a4b9-1757d50037bc"
},
{
"id": "d3a5161f-b466-4b2c-a7ad-0a954bc51645"
},
{
"id": "da383728-04f1-4eee-a0e1-882be4531df0"
},
{
"id": "6656e869-9660-4725-b872-54ceef21bdfa"
},
{
"id": "1756e8d5-dd7c-4921-9d3e-e8d57f402183"
},
{
"id": "4e4d876c-211f-47ec-8f20-687e2ea58dc9"
},
{
"id": "86dd560e-6cfc-47f0-b571-5377d72d1eb4"
},
{
"id": "a71916b4-c735-41d4-a80f-898a7a5f9c53"
},
{
"id": "60a5513d-fc6b-4fb6-8b19-e7adb72970e9"
},
{
"id": "6686e276-efbd-4393-8c6b-13ddd5b4ee4a"
}
],
"iconUrl": "https://api.seismic.com/icon-host/v1/content-icons-v2/icon?format=pptx",
"createdAt": "2024-03-01T20:08:13.283Z",
"createdBy": null,
"isContextualContent": null,
"modifiedAt": "2024-04-12T14:38:11.933Z",
"modifiedBy": null,
"resourceUrl": null,
"size": 16608126,
"urlObjectUrl": "https://demo.sharepoint.com/personal/jdemo_silvertower_co/_layouts/15/Doc.aspx?sourcedoc=%7BB87E1D7E-2491-4F46-8078-8F6E7723ACA6%7D&file=Brochure%20-%20SaaS%20Computing.pptx&action=edit&mobileredirect=true",
"workSpaceVersionType": "7",
"majorVersion": 3,
"minorVersion": 0,
"originUrl": null,
"deliveryOptionsDenyList": null
}
]
[
{
"contentTypeNumber": "6",
"selectedFrom": "library",
"repository": "library",
"id": "86e6f9ff-f60e-4b22-9a42-48fa2b29207a",
"name": "Cloud-Based Technology (SAAS)",
"type": "LiveDoc Component",
"format": "DOCX",
"description": null,
"sourceBlobId": "8aab3a90-48b0-4f92-9986-3b6c19a918f5",
"sourceContainerName": "sts",
"thumbnailId": "t4765de2f-9a30-41f6-98ae-aa78fbf463e1",
"thumbnailUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=sts&c=sts&id=t4765de2f-9a30-41f6-98ae-aa78fbf463e1&et=20240731051345&sig=demo%3D",
"sourceBlobDownloadUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=sts&c=sts&id=8aab3a90-48b0-4f92-9986-3b6c19a918f5&et=20240731051345&fn=8aab3a90-48b0-4f92-9986-3b6c19a918f5.DOCX&sig=demo%3D",
"versionId": "2215769d-9f7b-41d8-b19b-c3b828225607",
"teamsiteId": "60f938b7-0dcd-421a-81f9-ac89609317ef",
"version": "0.1",
"properties": [
{
"name": "Classification",
"id": "200bf162-a222-470b-aad7-12de91be911f",
"values": [
"Internal"
]
},
{
"name": "Asset Type",
"id": "ca305797-b572-4ec4-b487-35986cbadc50",
"values": [
"RFP"
]
},
{
"name": "Category",
"id": "9220fce8-5362-4e15-9f70-3844b089782a",
"values": [
"Product Functionality",
"About SilverTower"
]
},
{
"name": "Subject Matter",
"id": "53b5769c-a061-45b6-aa1a-37d703eac99f",
"values": [
"Analytics",
"Core Performance",
"Mobile",
"Diversified Alignment",
"Extended Efficiency"
]
}
],
"iconUrl": "https://api.seismic.com/icon-host/v1/content-icons-v2/icon?contentTypeNumber=6",
"createdAt": "2018-05-22T19:07:23.663Z",
"createdBy": null,
"size": 20656,
"urlObjectUrl": null,
"workSpaceVersionType": null,
"majorVersion": 0,
"minorVersion": 1,
"originUrl": null,
"deliveryOptionsDenyList": null
}
]
[
{
"contentTypeNumber": "3",
"selectedFrom": "recents",
"repository": "doccenter",
"id": "L2RkYzRmMDZhM2ItNDEwNS00ODY1LThlZjYtYWY5ZjJmOGVkYTJkL2xkMmMxYjk3MjgtMzA2My00ODFkLWI1OWYtMThiMTFhOGM2NGQ2L2xmNzRlZWJkNmUtYTVmMi00NzkwLWExNGYtMTY1NDkxNjdjZWVm",
"profileVersionId": "7af69b09-3ac0-4763-8672-48a13ffb7104",
"name": "5 Techniques For Handling Sales Objections",
"type": "file",
"format": "pptx",
"description": null,
"parentFolderId": "root",
"contentProfileId": "309ee9b8-235d-4ca9-907a-e06fec554058",
"sourceBlobId": null,
"sourceContainerName": "sts",
"contentProfileName": "Enterprise Sales - Français",
"path": [
{
"id": "ddc4f06a3b-4105-4865-8ef6-af9f2f8eda2d",
"name": "Sales Assets"
},
{
"id": "ld2c1b9728-3063-481d-b59f-18b11a8c64d6",
"name": "Internal Sales Training Resources"
},
{
"id": "lf74eebd6e-a5f2-4790-a14f-16549167ceef",
"name": "5 Techniques For Handling Sales Objections"
}
],
"thumbnailId": "tfabb7052-0426-4465-8560-17a5d7132420",
"thumbnailUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=sts&c=sts&id=tfabb7052-0426-4465-8560-17a5d7132420&et=20240731051807&sig=demo%3D",
"sourceBlobDownloadUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=sts&c=sts&id=a000c81f-2b2e-40c9-ad7e-7565aa16dad8&et=20240731051807&fn=a000c81f-2b2e-40c9-ad7e-7565aa16dad8.PPTX&sig=demo%3D",
"applicationUrls": [
{
"name": "DocCenter Universal Link",
"url": "https://sts.seismic.com/Link/Content/DCWVmDBWDEMODHPX2FP"
}
],
"libraryContent": {
"id": "34203a6b-eb14-4c23-86fa-7aa222a1cf1a",
"versionId": "74eebd6e-a5f2-4790-a14f-16549167ceef",
"teamsiteId": "1"
},
"expiresAt": null,
"properties": [],
"deliveryOptions": [],
"iconUrl": "https://api.seismic.com/icon-host/v1/content-icons-v2/icon?format=pptx",
"modifiedAt": "2019-09-10T18:11:02+00:00",
"size": "7850843",
"urlObjectUrl": null,
"workSpaceVersionType": null,
"majorVersion": null,
"minorVersion": null,
"originUrl": null,
"deliveryOptionsDenyList": [
"1756e8d5-dd7c-4921-9d3e-e8d57f402183",
"18f5165b-5136-4d31-b111-112e26cf2d7a",
"28d39fce-4a92-4267-a128-a355b1f95c2f",
"2d4227a5-eebc-4532-8e03-cf6f4b24427e",
"403f0842-3628-4524-bd0c-3bddac2d12cc",
"44bbcc3f-a458-4099-917d-64c7dcbc54eb",
"4e4d876c-211f-47ec-8f20-687e2ea58dc9",
"4f289f89-1261-4517-89b2-afa3140eeedd",
"579f59f3-99c5-46c3-a805-2391ca26b882",
"6686e276-efbd-4393-8c6b-13ddd5b4ee4a",
"6bf712d9-5e45-4beb-b913-0749372bb757",
"79705d28-1c9a-427f-994e-e9e963093b96",
"86dd560e-6cfc-47f0-b571-5377d72d1eb4",
"87ff95bc-4bfc-4d08-9c0f-a594acdb6530",
"c3991a94-5dc2-4488-adc4-46717669aa9c",
"cd448095-c4f9-4a25-a4b9-1757d50037bc",
"d3a5161f-b466-4b2c-a7ad-0a954bc51645",
"d8ddfdaa-5a07-455e-b797-bb12007568e6",
"da383728-04f1-4eee-a0e1-882be4531df0",
"dbd44b43-3d2a-4969-86e6-d61d0e079783",
"ec330dee-13c1-4dbd-8f4a-0777f4bfed16",
"ecf7a27f-87ee-41b7-812d-1655bbcb2fb0"
]
}
]
[
{
"contentTypeNumber": "3",
"selectedFrom": "favorites",
"repository": "doccenter",
"id": "L2RkOTA0ZGNlOTItNTAyMC1mYzc5LWUzMjAtMTkzYWFlOTE5N2IyL2xkZTI3ZTY4OWYtZjU2NC00ZDBiLTkxOWMtMjUyMDZmOTI2YzE3L2xkODY0Y2QwZjctMTY2ZC00NjcwLWFhNWQtYTRhZWJjZjM4ZDQxL2xmYmZiYmRkOWYtM2U4Zi00OTM1LTgxYWEtMjNjNWI0MTM0Mjll",
"profileVersionId": "8aecd097-18a9-477c-9b93-86e888926f17",
"name": "Diversified_Alignment_for_DEMO",
"type": "file",
"format": "pdf",
"description": null,
"parentFolderId": "root",
"contentProfileId": "0b0ed2d6-e3f2-452c-a217-8d1f79fc5eb9",
"sourceBlobId": null,
"sourceContainerName": "sts",
"contentProfileName": "Inside Sales - East",
"path": [
{
"id": "dd904dce92-5020-fc79-e320-193aae9197b2",
"name": "External Sync Content"
},
{
"id": "lde27e689f-f564-4d0b-919c-25206f926c17",
"name": "Dropbox"
},
{
"id": "ld864cd0f7-166d-4670-aa5d-a4aebcf38d41",
"name": "STS Sell Sheets"
},
{
"id": "lfbfbbdd9f-3e8f-4935-81aa-23c5b413429e",
"name": "Diversified_Alignment_for_CPG"
}
],
"thumbnailId": "t9091cb18-e92f-4853-92da-27924943ddec",
"thumbnailUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=sts&c=sts&id=t9091cb18-e92f-4853-92da-27924943ddec&et=20240731051917&sig=demo%3D",
"sourceBlobDownloadUrl": "https://newdownload.seismic.com/api/download/v1/blob?t=sts&c=sts&id=bcdd658b-2eb7-4c52-8bce-413a542300af&et=20240731051917&fn=bcdd658b-2eb7-4c52-8bce-413a542300af.PDF&sig=demo%3D",
"applicationUrls": [
{
"name": "DocCenter Universal Link",
"url": "https://sts.seismic.com/Link/Content/DCcXh9jDEMOmHGTf7MT7B"
}
],
"libraryContent": {
"id": "2c5eb7bb-09c9-4521-bf37-e948bccceb98",
"versionId": "bfbbdd9f-3e8f-4935-81aa-23c5b413429e",
"teamsiteId": "1"
},
"expiresAt": null,
"properties": [],
"deliveryOptions": [],
"iconUrl": "https://api.seismic.com/icon-host/v1/content-icons-v2/icon?format=pdf",
"modifiedAt": "2021-09-07T12:01:56+00:00",
"size": "274796",
"urlObjectUrl": null,
"workSpaceVersionType": null,
"majorVersion": null,
"minorVersion": null,
"originUrl": null,
"deliveryOptionsDenyList": []
}
]
Design
CSS Changes Not Supported
Future versions of the content picker will support CSS overrides. We have not yet locked down our class structure, so modify the CSS at your own risk.
Updated 6 months ago